Copyright (c) Hyperion Entertainment and contributors.
Graphics Primitives
![]() |
This page is currently being updated to AmigaOS 4.x. Some of the information contained here may not yet be applicable in part or totally. |
Graphics Primitives
This article describes the basic graphics functions available to Amiga programmers. It covers the graphics support structures, display routines and drawing routines. Many of the operations described in this section are also performed by the Intuition software. See the Intuition articles for more information.
The Amiga supports several basic types of graphics routines: display routines, drawing routines, sprites and animation. These routines are very versatile and allow you to define any combination of drawing and display areas you may wish to use.
This section explains all of the available modes of drawing supported by the system software, including how to do the following:
- Reserve memory space for use by the drawing routines.
- Define the colors that can be drawn into a drawing area.
- Define the colors of the drawing pens (foreground pen, background pen for patterns, and outline pen for area-fill outlines).
- Define the pen position in the drawing area.
- Drawing primitives; lines, rectangles, circles and ellipses.
- Define vertex points for area-filling, and specify the area-fill color and pattern.
- Define a pattern for patterned line drawing.
- Change drawing modes.
- Read or write individual pixels in a drawing area.
- Copy rectangular blocks of drawing area data from one drawing area to another.
- Use a template (predefined shape) to draw an object into a drawing area.
For those working with Classic Amiga displays such as ECS and AGA you will want to reference Classic Graphics Primitives.
Drawing Routines
Most of the graphics drawing routines require information about how the drawing is to take place. For this reason, most graphics drawing routines use a data structure called a RastPort, that contains pointers to the drawing area and drawing variables such as the current pen color and font to use. In general, you pass a pointer to your RastPort structure as an argument whenever you call a drawing function.
The RastPort Structure
The RastPort data structure can be found in the include files <graphics/rastport.h> and <graphics/rastport.i>. It contains the following information:
struct RastPort
{
struct Layer *Layer;
struct BitMap *BitMap;
UWORD *AreaPtrn; /* Ptr to areafill pattern */
struct TmpRas *TmpRas;
struct AreaInfo *AreaInfo;
struct GelsInfo *GelsInfo;
UBYTE Mask; /* Write mask for this raster */
BYTE FgPen; /* Foreground pen for this raster */
BYTE BgPen; /* Background pen */
BYTE AOlPen; /* Areafill outline pen */
BYTE DrawMode; /* Drawing mode for fill, lines, and text */
BYTE AreaPtSz; /* 2^n words for areafill pattern */
BYTE linpatcnt; /* Current line drawing pattern preshift */
BYTE dummy;
UWORD Flags; /* Miscellaneous control bits */
UWORD LinePtrn; /* 16 bits for textured lines */
WORD cp_x, cp_y; /* Current pen position */
UBYTE minterms[8];
WORD PenWidth;
WORD PenHeight;
struct TextFont *Font; /* Current font address */
UBYTE AlgoStyle; /* The algorithmically generated style */
UBYTE TxFlags; /* Text specific flags */
UWORD TxHeight; /* Text height */
UWORD TxWidth; /* Text nominal width */
UWORD TxBaseline; /* Text baseline */
WORD TxSpacing; /* Text spacing (per character) */
APTR *RP_User;
ULONG longreserved[2];
#ifndef GFX_RASTPORT_1_2
UWORD wordreserved[7]; /* Used to be a node */
UBYTE reserved[8]; /* For future use */
#endif
};
The sections that follow explain each of the items in the RastPort structure is used.
Initializing a RastPort Structure
Once you have a BitMap set up, you can declare and initialize the RastPort and then link the BitMap into it. Here is a sample initialization sequence:
struct BitMap bitMap = {0};
struct RastPort rastPort = {0};
/* Initialize the RastPort and link the BitMap to it. */
InitRastPort(&rastPort);
rastPort.BitMap = &bitMap;
Initialize, Then Link |
---|
You cannot link the bitmap in until after the RastPort has been initialized. |
RastPort Area-fill Information
Two structures in the RastPort–AreaInfo and TmpRas–define certain information for area filling operations. The AreaInfo pointer is initialized by a call to the routine InitArea().
#define AREA_SIZE 200
register USHORT i;
WORD areaBuffer[AREA_SIZE];
struct AreaInfo areaInfo = {0};
/* Clear areaBuffer before calling InitArea(). */
for (i=0; i < AREA_SIZE; i++)
areaBuffer[i] = 0;
InitArea(&areaInfo, areaBuffer, (AREA_SIZE*2)/5);
The area buffer must start on a word boundary. That is why the sample declaration shows areaBuffer as composed of unsigned words (200), rather than unsigned bytes (400). It still reserves the same amount of space, but aligns the data space correctly.
To use area fill, you must first provide a work space in memory for the system to store the list of points that define your area. You must allow a storage space of 5 bytes per vertex. To create the areas in the work space, you use the functions AreaMove(), AreaDraw(), and AreaEnd().
Typically, you prepare the RastPort for area-filling by following the steps in the code fragment above and then linking your AreaInfo into the RastPort like so:
rastPort->AreaInfo = &areaInfo;
In addition to the AreaInfo structure in the RastPort, you must also provide the system with some work space to build the object whose vertices you are going to define. This requires that you initialize a TmpRas structure, then point to that structure for your RastPort to use.
Here is a code fragment that builds and initializes a TmpRas. First the TmpRas structure is initialized (via InitTmpRas()) then it is linked into the RastPort structure.
Allocate Enough Space |
---|
The area to which TmpRas.RasPtr points must be at least as large as the area (width times height) of the largest rectangular region you plan to fill. Typically, you allocate a space as large as a single bitplane (usually 320*200 bits for Lores mode, 640*200 for Hires, and 1,280*200 for SuperHires). |
When you use functions that dynamically allocate memory from the system, you must remember to return these memory blocks to the system before your program exits. See the description of FreeRaster() in the SDK.
RastPort Graphics Element Pointer
The graphics element pointer in the RastPort structure is called GelsInfo.
If you are doing graphics animation using the GELS system, this pointer must refer to a properly initialized GelsInfo structure. See Graphics Sprites, Bobs and Animation for more information.
RastPort Write Mask
The write mask is a RastPort variable that determines which of the bitplanes are currently writable.
For most applications, this variable is set to all bits on. This means that all bitplanes defined in the BitMap are affected by a graphics writing operation. You can selectively disable one or more bitplanes by simply specifying a 0 bit in that specific position in the control byte. For example:
uint32 result = IGraphics->SetWriteMask(&rastPort, 0xFB); /* disable bitplane 2 */
if (result == 0)
IDOS->Printf("Can't set write mask\n");
A useful application for the Mask field is to set or clear plane 6 while in the Extra-Half-Brite display mode to create shadow effects. For example:
IGraphics->SetWriteMask(&rastPort, 0xE0); /* Disable planes 1 through 5. */
IGraphics->SetAPen(&rastPort, 0); /* Clear the Extra-Half-Brite bit */
IGraphics->RectFill(&rastPort, 20, 20, 40, 30); /* in the old rectangle. */
IGraphics->SetAPen(&rastPort, 32); /* Set the Extra-Half-Brite bit */
IGraphics->RectFill(&rastPort, 30, 25, 50, 35); /* in the new rectangle. */
IGraphics->SetWrMsk(&rastPort, -1); /* Re-enable all planes. */
Note |
---|
Do not use the obsolete SetWrMsk() macro in new code. |
RastPort Drawing Pens
The Amiga has three different drawing “pens” associated with the graphics drawing routines. These are:
- FgPen
- The foreground or primary drawing pen. For historical reasons, it is also called the A-Pen.
- BgPen
- The background or secondary drawing pen. For historical reasons, it is also called the B-Pen.
- AOlPen
- The area outline pen. For historical reasons, it is also called the O-Pen.
A drawing pen variable in the RastPort contains the current value (range 0-255) for a particular color choice. This value represents a color register number whose contents are to be used in rendering a particular type of image. The effect of the pen value is dependent upon the drawing mode and can be influenced by the pattern variables and the write mask as described below. Always use the system calls (e.g. SetAPen()) to set the different pens, never store values directly into the pen fields of the RastPort.
Colors Repeat Beyond 31 |
---|
The Amiga 500/2000/3000 (with original chips or ECS) contains only 32 color registers. Any range beyond that repeats the colors in 0-31. For example, pen numbers 32-63 refer to the colors in registers 0-31 (except when you are using Extra-Half-Brite mode). |
The graphics library drawing routines support BitMaps up to eight planes deep allowing for future expansion of the Amiga hardware.
The color in FgPen is used as the primary drawing color for rendering lines and areas. This pen is used when the drawing mode is JAM1 (see the next section for drawing modes). JAM1 specifies that only one color is to be “jammed” into the drawing area.
You establish the color for FgPen using the statement:
SetAPen(&rastPort, newcolor);
The color in BgPen is used as the secondary drawing color for rendering lines and areas. If you specify that the drawing mode is JAM2 (jamming two colors) and a pattern is being drawn, the primary drawing color (FgPen) is used where there are 1s in the pattern. The secondary drawing color (BgPen) is used where there are 0s in the pattern.
You establish the drawing color for BgPen using the statement:
SetBPen(&rastPort, newcolor);
The area outline pen AOlPen is used in two applications: area fill and flood fill. (See “Area Fill Operations” below.) In area fill, you can specify that an area, once filled, can be outlined in this AOlPen color. In flood fill (in one of its operating modes) you can fill until the flood-filler hits a pixel of the color specified in this pen variable.
You establish the drawing color for AOlPen using the statement:
SetOutlinePen(&rastPort, newcolor);
Note |
---|
Do not use the obsolete SetOPen() macro. |
RastPort Drawing Modes
Four drawing modes may be specified in the RastPort.DrawMode field:
- JAM1
- Whenever you execute a graphics drawing command, one color is jammed into the target drawing area. You use only the primary drawing pen color, and for each pixel drawn, you replace the color at that location with the FgPen color.
- JAM2
- Whenever you execute a graphics drawing command, two colors are jammed into the target drawing area. This mode tells the system that the pattern variables (both line pattern and area pattern – see the next section) are to be used for the drawing. Wherever there is a 1 bit in the pattern variable, the FgPen color replaces the color of the pixel at the drawing position. Wherever there is a 0 bit in the pattern variable, the BgPen color is used.
- COMPLEMENT
- For each 1 bit in the pattern, the corresponding bit in the target area is complemented – that is, its state is reversed. As with all other drawing modes, the write mask can be used to protect specific bitplanes from being modified. Complement mode is often used for drawing and then erasing lines.
- INVERSVID
- This is the drawing mode used primarily for text. If the drawing mode is (JAM1 | INVERSVID), the text appears as a transparent letter surrounded by the FgPen color. If the drawing mode is (JAM2|INVERSVID), the text appears as in (JAM1|INVERSVID) except that the BgPen color is used to draw the text character itself. In this mode, the roles of FgPen and BgPen are effectively reversed.
You set the drawing modes using the statement:
SetDrMd(&rastPort, newmode);
Set the newmode argument to one of the four drawing modes listed above.
RastPort Line and Area Drawing Patterns
The RastPort data structure provides two different pattern variables that it uses during the various drawing functions: a line pattern and an area pattern.
The line pattern is 16 bits wide and is applied to all lines. When you initialize a RastPort, this line pattern value is set to all 1s (hex FFFF), so that solid lines are drawn. You can also set this pattern to other values to draw dotted lines if you wish.
For example, you can establish a dotted line pattern with the graphics macro SetDrPt():
SetDrPt(&rastPort, 0xCCCC);
The second argument is a bit-pattern, 1100110011001100, to be applied to all lines drawn. If you draw multiple, connected lines, the pattern cleanly connects all the points.
The area pattern is also 16 bits wide and its height is some power of two. This means that you can define patterns in heights of 1, 2, 4, 8, 16, and so on. To tell the system how large a pattern you are providing, use the graphics macro SetAfPt():
SetAfPt(&rastPort, &areaPattern, power_of_two);
The &areaPattern argument is the address of the first word of the area pattern and power_of_two specifies how many words are in the pattern. For example:
uint16 ditherData[] =
{
0x5555, 0xAAAA
};
SetAfPt(&rastPort, ditherData, 1);
This example produces a small cross-hatch pattern, useful for shading. Because power_of_two is set to 1, the pattern height is 2 to the 1st, or 2 rows high.
To clear the area fill pattern, use:
SetAfPt(&rastPort, NULL, 0);
Pattern Positioning |
---|
The pattern is always positioned with respect to the upper left corner of the RastPort drawing area (the 0,0 coordinate). If you draw two rectangles whose edges are adjacent, the pattern will be continuous across the rectangle boundaries. |
The last example given produces a two-color pattern with one color where there are 1s and the other color where there are 0s in the pattern. A special mode allows you to develop a pattern having up to 256 colors. To create this effect, specify power_of_two as a negative value instead of a positive value. For instance, the following initialization establishes an 8-color checkerboard pattern where each square in the checkerboard has a different color.
uint16 areaPattern[3][8] =
{
/* plane 0 pattern */
{
0x0000, 0x0000,
0xffff, 0xffff,
0x0000, 0x0000,
0xffff, 0xffff
},
/* plane 1 pattern */
{
0x0000, 0x0000,
0x0000, 0x0000,
0xffff, 0xffff,
0xffff, 0xffff
},
/* plane 2 pattern */
{
0xff00, 0xff00,
0xff00, 0xff00,
0xff00, 0xff00,
0xff00, 0xff00
}
};
SetAfPt(&rastPort, areaPattern, -3);
/* when doing this, it is best to set three other parameters as follows: */
SetAPen(&rastPort, -1);
SetBPen(&rastPort, 0);
SetDrMd(&rastPort, JAM2);
If you use this multicolored pattern mode, you must provide as many planes of pattern data as there are planes in your BitMap.
RastPort Pen Position and Size
The graphics drawing routines keep the current position of the drawing pen in the RastPort fields cp_x and cp_y, for the horizontal and vertical positions, respectively.
The coordinate location 0,0 is in the upper left corner of the drawing area. The x value increases proceeding to the right; the y value increases proceeding toward the bottom of the drawing area.
The variables RastPort.PenWidth and RastPort.PenHeight are not currently implemented. These fields should not be read or written by applications.
Text Attributes
Text attributes and font information are stored in the RastPort fields Font, AlgoStyle, TxFlags, TxHeight, TxWidth, TxBaseline and TxSpacing. These are normally set by calls to the graphics font routines which are covered separately in Graphics Library and Text.
Working with 32 bit colours on direct mapped screens
As well as the individual functions for setting the various RastPort attributes (e.g. SetAPen(), SetBPen()) you can also use the function SetRPAttrs(). SetRPAttrs() also allows you to define multiple attributes in a single call using a tag list.
When working with 32 bit colour values use SetRPAttrs() with the tags RPTAG_APenColor, RPTAG_BPenColor and RPTAG_OPenColor. This of course only works on direct mapped bitmaps (e.g. 16bit or 32bit).
The colour format used by the three tags above is 0xAARRGGBB. The alpha part is currently ignored and should be set to 0xFF so that the correct alpha is used when alpha blending has been implemented.
The following code snippet might be used to render a light blue and yellow dotted line using 32bit colours.
IGraphics->SetRPAttrs(rp,
RPTAG_APenColor, 0xFF9999FF, // light blue
RPTAG_BPenColor, 0xFFFFFF00, // yellow
RPTAG_DrMd, JAM2, // use two colours
TAG_END);
SetDrPt(rp, 0xFF00); // NB this one is a macro so no 'IGraphics'
IGraphics->Move(rp, x1, y1);
IGraphics->Draw(rp, x2, y2);
Using the Graphics Drawing Routines
This section shows you how to use the Amiga drawing routines. All of these routines work either on their own or along with the windowing system and layers library. For details about using the layers and windows, see Layers Library and Intuition Windows.
Use WaitBlit() |
---|
The graphics library rendering and data movement routines generally wait to get access to the blitter, start their blit, and then exit. Therefore, you must WaitBlit() after a graphics rendering or data movement call if you intend to immediately deallocate, examine, or perform order-dependent processor operations on the memory used in the call. |
As you read this section, keep in mind that to use the drawing routines, you need to pass them a pointer to a RastPort. You can define the RastPort directly, as shown in the sample program segments in preceding sections, or you can get a RastPort from your Window structure using code like the following:
struct Window *window;
struct RastPort *rastPort;
window = IIntuition->OpenWindow(&newWindow); /* You could use OpenWindowTags() instead. */
if (window)
rastPort = window->RPort;
You can also get the RastPort from the Layer structure, if you are not using Intuition.
Drawing Individual Pixels
You can set a specific pixel to a desired color by using a statement like this:
int16 x, y;
int32 result = IGraphics->WritePixel(&rastPort, x, y);
WritePixel() uses the primary drawing pen and changes the pixel at that x,y position to the desired color if the x,y coordinate falls within the boundaries of the RastPort. A value of 0 is returned if the write was successful; a value of -1 is returned if x,y was outside the range of the RastPort.
Reading Individual Pixels
You can determine the color of a specific pixel with a statement like this:
int16 x, y;
int32 result = IGraphics->ReadPixel(&rastPort, x, y);
ReadPixel() returns the value of the pixel color selector at the specified x,y location. If the coordinates you specify are outside the range of your RastPort, this function returns a value of -1.
Drawing Ellipses and Circles
Two functions are associated with drawing ellipses: DrawCircle() and DrawEllipse(). DrawCircle(), a macro that calls DrawEllipse(), will draw a circle from the specified center point using the specified radius. This function is executed by the statement:
DrawCircle(&rastPort, center_x, center_y, radius);
Similarly, DrawEllipse() draws an ellipse with the specified radii from the specified center point:
DrawEllipse(&rastPort, center_x, center_y, horiz_r, vert_r);
Neither function performs clipping on a non-layered RastPort.
Drawing Lines
Two functions are associated with line drawing: Move() and Draw().
Move() simply moves the cursor to a new position. It is like picking up a drawing pen and placing it at a new location. This function is executed by the statement:
Move(&rastPort, x, y);
Draw() draws a line from the current x,y position to a new x,y position specified in the statement itself. The drawing pen is left at the new position. This is done by the statement:
Draw(&rastPort, x, y);
Draw() uses the pen color specified for FgPen.
Here is a sample sequence that draws a line from location (0,0) to (100,50).
IGraphics->SetAPen(&rastPort, COLOR1); /* Set A pen color. */
IGraphics->Move(&rastPort, 0, 0); /* Move to this location. */
IGraphics->Draw(&rastPort, 100, 50); /* Draw to a this location. */
Caution |
---|
If you attempt to draw a line outside the bounds of the BitMap, using the basic initialized RastPort, you may crash the system. You must either do your own software clipping to assure that the line is in range, or use the layers library. Software clipping means that you need to determine if the line will fall outside your BitMap before you draw it, and render only the part which falls inside the BitMap. |
Drawing Patterned Lines
To turn the example above into a patterned line draw, simply set a drawing pattern, such as:
SetDrPt(&rastPort, 0xAAAA);
Now all lines drawn appear as dotted lines (0xAAAA = 1010101010101010 in binary). To resume drawing solid lines, execute the statement:
SetDrPt(&rastPort, ~0);
Because ~0 is defined as all bits on (11...11) in binary.
Drawing Multiple Lines with a Single Command
You can use multiple Draw() statements to draw connected line figures.
If the shapes are all definable as interconnected, continuous lines, you can use a simpler function, called PolyDraw(). PolyDraw() takes a set of line endpoints and draws a shape using these points. You call PolyDraw() with the statement:
PolyDraw(&rastPort, count, arraypointer);
PolyDraw() reads the array of points and draws a line from the first pair of coordinates to the second, then a connecting line to each succeeding pair in the array until count points have been connected. This function uses the current drawing mode, pens, line pattern, and write mask specified in the target RastPort; for example, this fragment draws a rectangle, using the five defined pairs of x,y coordinates.
int16 linearray[] =
{
3, 3,
15, 3,
15,15,
3,15,
3, 3
};
IGraphics->PolyDraw(&rastPort, 5, linearray);
Area-fill Operations
Assuming that you have properly initialized your RastPort structure to include a properly initialized AreaInfo, you can perform area fill by using the functions described in this section.
AreaMove() tells the system to begin a new polygon, closing off any other polygon that may already be in process by connecting the end-point of the previous polygon to its starting point.
AreaMove() is executed with the statement:
int32 result = IGraphics->AreaMove(&rastPort, x, y);
AreaMove() returns 0 if successful, -1 if there was no more space left in the vector list.
AreaDraw() tells the system to add a new vertex to a list that it is building. No drawing takes place until AreaEnd() is executed. AreaDraw is executed with the statement:
int32 result = IGraphics->AreaDraw(&rastPort, x, y);
AreaDraw() returns 0 if successful, -1 if there was no more space left in the vector list.
AreaEnd() tells the system to draw all of the defined shapes and fill them. When this function is executed, it obeys the drawing mode and uses the line pattern and area pattern specified in your RastPort to render the objects you have defined.
To fill an area, you do not have to AreaDraw() back to the first point before calling AreaEnd(). AreaEnd() automatically closes the polygon. AreaEnd() is executed with the following statement:
int32 result = AreaEnd(&rastPort);
AreaEnd() returns 0 if successful, -1 if there was an error.
To turn off the outline function, you have to set the RastPort Flags variable back to 0 with BNDRYOFF():
#include <graphics/gfxmacros.h>
BNDRYOFF(&rastPort);
Otherwise, every subsequent area-fill or rectangle-fill operation will outline their rendering with the outline pen (AOlPen).
Ellipse and Circle-fill Operations
Two functions are associated with drawing filled ellipses: AreaCircle() and AreaEllipse(). AreaCircle() (a macro that calls AreaEllipse()) will draw a circle from the specified center point using the specified radius. This function is executed by the statement:
IGraphics->AreaCircle(&rastPort, center_x, center_y, radius);
Similarly, AreaEllipse() draws a filled ellipse with the specified radii from the specified center point:
IGraphics->AreaEllipse(&rastPort, center_x, center_y, horiz_r, vert_r);
Outlining with SetOutlinePen() is not currently supported by the AreaCircle() and AreaEllipse() routines.
Caution |
---|
If you attempt to fill an area outside the bounds of the BitMap, using the basic initialized RastPort, it may crash the system. You must either do your own software clipping to assure that the area is in range, or use the layers library. |
Flood-fill Operations
Flood fill is a technique for filling an arbitrary shape with a color. The Amiga flood-fill routines can use a plain color or do the fill using a combination of the drawing mode, FgPen, BgPen and the area pattern.
Flood-fill requires a TmpRas structure at least as large as the RastPort in which the flood-fill will be done. This is to ensure that even if the flood-filling operation “leaks”, it will not flow outside the TmpRas and corrupt another task’s memory.
You use the Flood() routine for flood fill. The syntax for this routine is as follows:
IGraphics->Flood(&rastPort, mode, x, y);
The rastPort argument specifies the RastPort you want to draw into. The x and y arguments specify the starting coordinate within the BitMap. The mode argument tells how to do the fill. There are two different modes for flood fill:
In outline mode, you specify an x,y coordinate, and from that point the system searches outward in all directions for a pixel whose color is the same as that specified in the area outline pen (AOlPen). All horizontally or vertically adjacent pixels not of that color are filled with a colored pattern or plain color. The fill stops at the outline color. Outline mode is selected when the mode argument to Flood() is set to a 0.
In color mode, you specify an x,y coordinate, and whatever pixel color is found at that position defines the area to be filled. The system searches for all horizontally or vertically adjacent pixels whose color is the same as this one and replaces them with the colored pattern or plain color. Color mode is selected when the mode argument to Flood() is set to a one.
The following sample program fragment creates and then flood-fills a triangular region. The overall effect is exactly the same as shown in the preceding area-fill example above, except that flood-fill is slightly slower than area-fill. Mode 0 (fill to a pixel that has the color of the outline pen) is used in the example.
int8 oldAPen;
uint16 oldDrPt;
struct RastPort *rastPort = Window->RPort;
/* Save the old values of the foreground pen and draw pattern. */
oldAPen = rastPort->FgPen;
oldDrPt = rastPort->LinePtrn;
/* Use AreaOutline pen color for foreground pen. */
IGraphics->SetAPen(rastPort, rastPort->AOlPen);
IGraphics->SetDrPt(rastPort, ~0); /* Insure a solid draw pattern. */
IGraphics->Move(rastPort, 0, 0); /* Using mode 0 to create a triangular shape */
IGraphics->Draw(rastPort, 0, 100);
IGraphics->Draw(rastPort, 100, 100);
IGraphics->Draw(rastPort, 0, 0); /* close it */
IGraphics->SetAPen(rastPort, oldAPen); /* Restore original foreground pen. */
IGraphics->Flood(rastPort, 0, 10, 50); /* Start Flood() inside triangle. */
SetDrPt(rastPort, oldDrPt); /* Restore original draw mode. */
This example saves the current FgPen value and draws the shape in the same color as AOlPen.
Then FgPen is restored to its original color so that FgPen, BgPen, DrawMode, and AreaPtrn can be used to define the fill within the outline.
Rectangle-fill Operations
The final fill function, RectFill(), is for filling rectangular areas. The form of this function follows:
RectFill(&rastPort, xmin, ymin, xmax, ymax);
As usual, the rastPort argument specifies the RastPort you want to draw into. The xmin and ymin arguments specify the upper left corner of the rectangle to be filled. The xmax and ymax arguments specify the lower right corner of the rectangle to be filled. Note that the variable xmax must be equal to or greater than xmin, and ymax must be equal to or greater than ymin.
Rectangle-fill uses FgPen, BgPen, AOlPen, DrawMode, AreaPtrn and Mask to fill the area you specify.
Remember that the fill can be multicolored as well as single- or two-colored. When the DrawMode is COMPLEMENT, it complements all bit planes, rather than only those planes in which the foreground is non-zero.
Performing Data Move Operations
The graphics library includes several routines that use the hardware blitter to handle the rectangularly organized data that you work with when doing raster-based graphics. These blitter routines do the following:
- Clear an entire segment of memory
- Set a raster to a specific color
- Scroll a subrectangle of a raster
- Draw a pattern "through a stencil"
- Extract a pattern from a bit-packed array and draw it into a raster
- Copy rectangular regions from one bitmap to another
- Control and utilize the hardware-based data mover, the blitter
The following sections cover these routines in detail.
Warning |
---|
The graphics library rendering and data movement routines generally wait to get access to the blitter, start their blit, and then exit without waiting for the blit to finish. Therefore, you must WaitBlit() after a graphics rendering or data movement call if you intend to immediately deallocate, examine, or perform order-dependent processor operations on the memory used in the call. |
Clearing a Memory Area
For memory that is accessible to the blitter (that is, internal Chip memory), the most efficient way to clear a range of memory is to use the blitter. You use the blitter to clear a block of memory with the statement:
BltClear(memblock, bytecount, flags);
The memblock argument is a pointer to the location of the first byte to be cleared and bytecount is the number of bytes to set to zero. In general the flags variable should be set to one to wait for the blitter operation to complete. Refer to the SDK for other details about the flag argument.
Setting a Whole Raster to a Color
You can preset a whole raster to a single color by using the function SetRast().
A call to this function takes the following form:
SetRast(&rastPort, pen);
As always, the &rastPort is a pointer to the RastPort you wish to use. Set the pen argument to the color register you want to fill the RastPort with.
Scrolling a Sub-rectangle of a Raster
You can scroll a sub-rectangle of a raster in any direction–up, down, left, right, or diagonally.
To perform a scroll, you use the ScrollRaster() routine and specify a dx and dy (delta-x, delta-y) by which the rectangle image should be moved relative to the (0,0) location.
As a result of this operation, the data within the rectangle will become physically smaller by the size of delta-x and delta-y, and the area vacated by the data when it has been cropped and moved is filled with the background color (color in BgPen). ScrollRaster() is affected by the Mask setting.
Here is the syntax of the ScrollRaster() function:
ScrollRaster(&rastPort, dx, dy, xmin, ymin, xmax, ymax);
The &rastPort argument is a pointer to a RastPort. The dx and dy arguments are the distances (positive, 0, or negative) to move the rectangle. The outer bounds of the sub-rectangle are defined by the xmin, xmax, ymin and ymax arguments.
Here are some examples that scroll a sub-rectangle:
/* scroll up 2 */
ScrollRaster(&rastPort, 0, 2, 10, 10, 50, 50);
/* scroll right 1 */
ScrollRaster(&rastPort, -1, 0, 10, 10, 50, 50);
When scrolling a Simple Refresh window (or other layered RastPort), ScrollRaster() scrolls the appropriate existing damage region. Refer to Intuition Windows for an explanation of Simple Refresh windows and damage regions.
When scrolling a SuperBitMap window ScrollRaster() requires a properly initialized TmpRas. The TmpRas must be initialized to the size of one bitplane with a width and height the same as the SuperBitMap, using the technique described in the "Area-Fill Information" section above.
If you are using a SuperBitMap Layer, it is possible that the information in the BitMap is not fully reflected in the layer and vice-versa. Two graphics calls, CopySBitMap() and SyncSBitMap(), remedy these situations. Again, refer to Intuition Windows for more on this.
Drawing through a Stencil
The routine BltPattern() allows you to change only a very selective portion of a drawing area.
Basically, this routine lets you define a rectangular region to be affected by a drawing operation and a mask of the same size that further defines which pixels within the rectangle will be affected.
The figure below shows an example of what you can do with BltPattern(). The 0 bits are represented by blank rectangles; the 1 bits by filled-in rectangles.
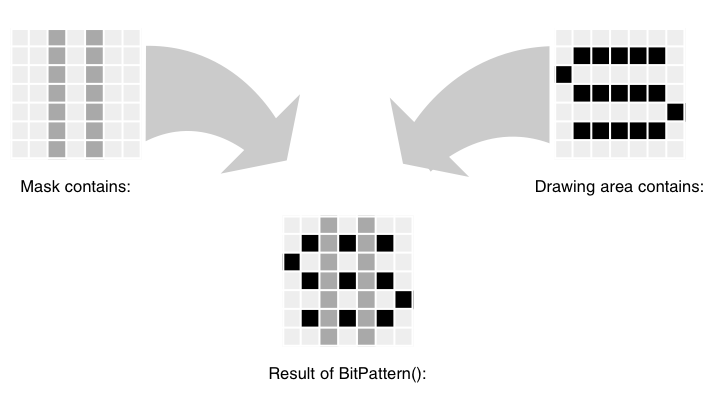
In the resulting drawing, the lighter squares show where the target drawing area has been affected. Exactly what goes into the drawing area when the mask has 1’s is determined by your RastPort’s FgPen, BgPen, DrawMode and AreaPtrn fields.
You call BltPattern() with:
BltPattern(&rastport, mask, xl, yl, maxx, maxy, bytecnt)
The &rastport argument specifies the RastPort to use. The operation will be confined to a rectangular area within the RastPort specified by xl and yl (upper right corner of the rectangle) and maxx and maxy (lower right corner of the rectangle).
The mask is a pointer to the mask to use. This can be NULL, in which case a simple rectangular region is modified. Or it can be set to the address of a byte pattern which allows any arbitrary shape within the rectangle to be defined. The bytecount is the number of bytes per row for the mask (it must be an even number of bytes).
The mask parameter is a rectangularly organized, contiguously stored pattern. This means that the pattern is stored in sequential memory locations stored as (maxy - yl + 1) rows of bytecnt bytes per row. These patterns must obey the same rules as BitMaps. This means that they must consist of an even number of bytes per row and must be stored in memory beginning at a legal word address. (The mask for BltPattern() does not have to be in Chip RAM, though.)
Extracting from a Bit-packed Array
You use the routine BltTemplate() to extract a rectangular area from a source area and place it into a destination area.
The following figure shows an example.
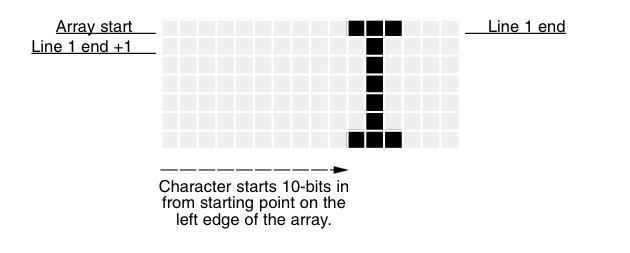
For a rectangular bit array to be extracted from within a larger, rectangular bit array, the system must know how the larger array is organized. For this extraction to occur properly, you also need to tell the system the modulo for the inner rectangle.
The modulo is the value that must be added to the address pointer so that it points to the correct word in the next line in this rectangularly organized array.
The following figure represents a single bitplane and the smaller rectangle to be extracted.
The modulo in this instance is 4, because at the end of each line, you must add 4 to the address pointer to make it point to the first word in the smaller rectangle.
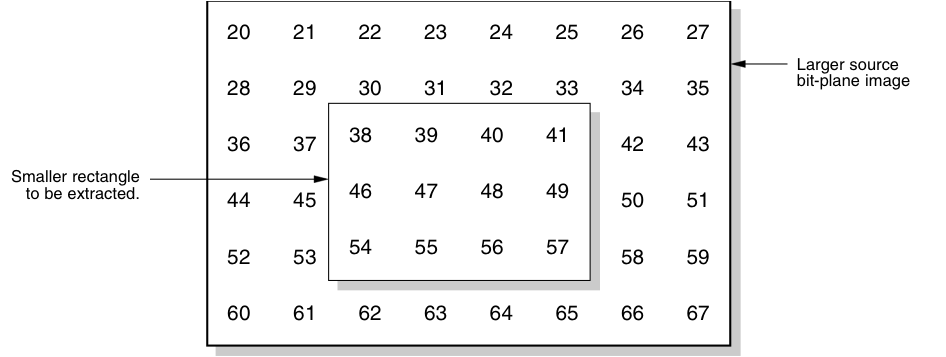
Warning |
---|
The modulo value must be an even number of bytes. |
BltTemplate() takes the following arguments:
BltTemplate(source, srcX, srcMod, &destRastPort, destX, destY, sizeX, sizeY);
The source argument specifies the rectangular bit array to use as the source template. Set this to the address of the nearest word (rounded down) that contains the first line of the source rectangle. The srcX argument gives the exact bit position (0-15) within the source address at which the rectangular bit array begins. The srcMod argument sets the source modulo so the next line of the rectangular bit array can be found.
The data from the source rectangle is copied into the destination RastPort specified by destRastPort. The destX and destY arguments indicate where the data from the source rectangle should be positioned within the destination RastPort. The sizeX and sizeY arguments indicate the dimensions of the data to be moved.
BltTemplate() uses FgPen, BgPen, DrawMode and Mask to place the template into the destination area.
This routine differs from BltPattern() in that only a solid color is deposited in the destination drawing area, with or without a second solid color as the background (as in the case of text). Also, the template can be arbitrarily bit-aligned and sized in x.
Copying Rectangular Areas
Need BltBitMapTagList() documentation added here.
Four routines use the blitter to copy rectangular areas from one section of a BitMap to another: BltBitMap(), BltMaskBitMapRastPort(), BltBitMapRastPort() and ClipBlit(). All four of these blitter routines take a special argument called a minterm.
The minterm variable is an unsigned byte value which represents an action to be performed during the move. Since all the blitter routines uses the hardware blitter to move the data, they can take advantage of the blitter’s ability to logically combine or change the data as the move is made. The most common operation is a direct copy from source area to destination, which uses a minterm set to hex value C0.
You can determine how to set the minterm variable by using the logic equations shown in the following tables. B represents data from the source rectangle and C represents data in the destination area.
Leftmost 4 Bits of MinTerm | Logic Term Included in Final Output |
---|---|
8 | BC "B AND C" |
4 | B!C "B AND NOT C" |
2 | !BC "NOT B AND C" |
1 | !B!C "NOT B AND NOT C" |
You can combine values to select the logic terms. For instance a minterm value of 0xC0 selects the first two logic terms in the table above. These logic terms specify that in the final destination area you will have data that occurs in source B only. Thus, C0 means a direct copy. The logic equation for this is:
BC + B!C = B(C + !C) = B
Logic equations may be used to decide on a number of different ways of moving the data. For your convenience, a few of the most common ones are listed below.
Minterm Value | Logic Operation Performed During Copy |
---|---|
30 | Replace destination area with inverted source B. |
50 | Replace destination area with an inverted version of itself. |
60 | Put B where C is not, put C where B is not (cookie cut). |
80 | Only put bits into destination where there is a bit in the same position for both source and destination (sieve operation). |
C0 | Plain vanilla copy from source B to destination C. |
The graphics library blitter routines all accept a minterm argument as described above. BltBitMap() is the basic blitter routine, moving data from one BitMap to another.
BltBitMap() allows you to define a rectangle within a source BitMap and copy it to a destination area of the same size in another (or even the same) BitMap. This routine is used by the graphics library itself for rendering. BltBitMap() returns the number of planes actually involved in the blit. The syntax for the function is:
uint32 planes;
planes = BltBitMap(&srcBM, srcX, srcY, &dstBM, dstX, dstY,
sizeX, sizeY, minterm, mask, tempA);
The source bitmap is specified by the &srcBM argument. The position of the source area within the bitmap is specified by srcX and srcY. The destination bitmap is specified by the &dstBM argument. The position of the destination area within the bitmap is specified by dstX and dstY.
The dimensions (in pixels) of the area to be moved is indicated by the sizeX and sizeY arguments. With the original custom chip set, the blitter size limits are 992 x 1,024. With ECS the blitter size limits are 32,736 x 32,768. See the section on Determining Chip Versions to find out how to tell if the host system has ECS installed.
The minterm argument determines what logical operation to perform on the rectangle data as bits are moved (described above). The mask argument, normally set to 0xff, specifies which bitplanes will be involved in the blit operation and which will be ignored. If a bit is set in the mask byte, the corresponding bitplane is included. The tempA argument applies only to blits that overlap and, if non-NULL, points to Chip memory the system will use for temporary storage during the blit.
BltBitMapRastPort() takes most of the same arguments as BltBitMap(), but its destination is a RastPort instead of a BitMap. The syntax for the function is:
VOID BltBitMapRastPort(&srcBM, srcX, srcY, &dstRP, dstX, dstY,
sizeX, sizeY, minterm);
The arguments here are the same as for BltBitMap() above. Note that the BltBitMapRastPort() function will respect the RastPort.Mask field. Only the planes specified in the Mask will be included in the operation.
A third type of blitter operation is provided by the BltMaskBitMapRastPort() function. This works the same as BltBitMapRastPort() except that it takes one extra argument, a pointer to a single bitplane mask of the same height and width as the source. The mask acts as a filter for the operation–a blit only occurs where the mask plane is non-zero. The syntax for the function is:
VOID BltMaskBitMapRastPort(&srcBM, srcX, srcY, &dstRP, dstX, dstY,
sizeX, sizeY, minterm, bltmask);
The bltmask argument points to a word-aligned mask bitplane in Chip memory with the same dimensions as the source bitmap. Note that this function ignores the Mask field of the destination RastPort.
ClipBlit() takes most of the same arguments as the other blitter calls described above but it works with source and destination RastPorts and their layers. Before ClipBlit() moves data, it looks at the area from which and to which the data is being copied (RastPorts, not BitMaps) and determines if there are overlapping areas involved. If so, it splits up the overall operation into a number of bitmaps to move the data in the way you request. To call ClipBlit() use:
VOID ClipBlit(&srcRP, srcX, srcY, &dstRP, dstX, dstY, XSize, YSize, minterm);
Since ClipBlit() respects the Layer of the source and destination RastPort, it is the easiest blitter movement call to use with Intuition windows. The following code fragments show how to save and restore an undo buffer using ClipBlit().
/* Save work rastport to an undo rastport */
ClipBlit(&drawRP, 0, 0, &undoRP, 0, 0, areaWidth, areaHeight, 0xC0);
/* restore undo rastport to work rastport */
ClipBlit(&undoRP, 0, 0, &drawRP, 0, 0, areaWidth, areaHeight, 0xC0);
Scaling Rectangular Areas
BitMapScale() will scale a single bitmap any integral size up to 16,383 times its original size. It is called with the address of a BitScaleArgs structure (see <graphics/scale.h>).
VOID BitMapScale(struct BitScaleArgs *bsa);
The bsa argument specifies the BitMaps to use, the source and destination rectangles, as well as the scaling factor. The source and destination may not overlap. The caller must ensure that the destination BitMap is large enough to receive the scaled-up copy of the source rectangle. The function ScalerDiv() is provided to help in the calculation of the destination BitMap’s size.
When to Wait for the Blitter
This section explains why you might have to call WaitBlit(), a special graphics function that suspends your task until the blitter is idle. Many of the calls in the graphics library use the Amiga’s hardware blitter to perform their operation, most notably those which render text and images, fill or pattern, draw lines or dots and move blocks of graphic memory.
Internally, these graphics library functions operate in a loop, doing graphic operations with the blitter one plane at a time as follows:
IGraphics->OwnBlitter(); /* Gain exclusive access to the hardware blitter */
for(planes=0; planes < bitmap->depth; planes++)
{
IGraphics->WaitBlit(); /* Sleep until the previous blitter operation completes */
/* start a blit */
}
IGraphics->DisownBlitter(); /* Release exclusive access to the hardware blitter */
Graphics library functions that are implemented this way always wait for the blitter at the start and exit right after the final blit is started. It is important to note that when these blitter-using functions return to your task, the final (or perhaps only) blit has just been started, but not necessarily completed. This is efficient if you are making many such calls in a row because the next graphics blitter call always waits for the previous blitter operation to complete before starting its first blit.
However, if you are intermixing such graphics blitter calls with other code that accesses the same graphics memory then you must first WaitBlit() to make sure that the final blit of a previous graphics call is complete before you use any of the memory. For instance, if you plan to immediately deallocate or reuse any of the memory areas which were passed to your most recent blitter-using function call as a source, destination, or mask, it is imperative that you first call WaitBlit().
Warning |
---|
If you do not follow the above procedure, you could end up with a program that works correctly most of the time but crashes sometimes. Or you may run into problems when your program is run on faster machines or under other circumstances where the blitter is not as fast as the processor. |
Function Reference
The following are brief descriptions of the Amiga’s graphics primitives. See the SDK for details on each function call.
Function | Description |
---|---|
InitView() | Initializes the View structure. |
InitBitMap() | Initializes the BitMap structure. |
RASSIZE() | Calculates the size of a ViewPort’s BitMap. |
AllocRaster() | Allocates the bitplanes needed for a BitMap. |
FreeRaster() | Frees the bitplanes created with AllocRaster(). |
InitVPort() | Initializes the ViewPort structure. |
GetColorMap() | Returns the ColorMap structure used by ViewPorts. |
FreeColorMap() | Frees the ColorMap created by GetColorMap(). |
LoadRGB4() | Loads the color registers for a given ViewPort. |
SetRGB4CM() | Loads an individual color register for a given ViewPort. |
MakeVPort() | Creates the intermediate Copper list program for a ViewPort. |
MrgCop() | Merges the intermediate Copper lists. |
LoadView() | Displays a given View. |
FreeCprList() | Frees the Copper list created with MrgCop() |
FreeVPortCopLists() | Frees the intermediate Copper lists created with MakeVPort(). |
OFF_DISPLAY | Turns the video display DMA off |
ON_DISPLAY | Turns the video display DMA back on again. |
FindDisplayInfo() | Returns the display database handle for a given ModeID. |
GetDisplayInfoData() | Looks up a display attribute in the display database. |
VideoControl() | Sets, clears and gets the attributes of an existing display. |
GfxNew() | Creates ViewExtra or ViewPortExtra used in displays. |
GfxAssociate() | Attaches a ViewExtra to a View. |
GfxFree() | Frees the ViewExtra or ViewPortExtra created by GfxNew(). |
OpenMonitor() | Returns the MonitorSpec structure used in Views. |
CloseMonitor() | Frees the MonitorSpec structure created by OpenMonitor(). |
GetVPModeID() | Returns the ModeID of an existing ViewPort. |
ModeNotAvailable() | Determines if a display mode is available from a given ModeID. |
Function | Description |
---|---|
InitRastPort() | Initialize a RastPort structure. |
InitArea() | Initialize the AreaInfo structure used with a RastPort. |
SetWrMask() | Set the RastPort.Mask. |
SetAPen() | Set the RastPort.FgPen foreground pen color. |
SetBPen() | Set the RastPort.BgPen background pen color. |
SetOPen() | Set the RastPort.AOlPen area fill outline pen color. |
SetDrMode() | Set the RastPort.DrawMode drawing mode. |
SetDrPt() | Set the RastPort.LinePtrn line drawing pattern. |
SetAfPt() | Set the RastPort area fill pattern and size. |
WritePixel() | Draw a single pixel in the foreground color at a given coordinate. |
ReadPixel() | Find the color of the pixel at a given coordinate. |
DrawCircle() | Draw a circle with a given radius and center point. |
DrawEllipse() | Draw an ellipse with the given radii and center point. |
Move() | Move the RastPort drawing pen to a given coordinate. |
Draw() | Draw a line from the current pen location to a given coordinate. |
PolyDraw() | Draw a polygon with a given set of vertices. |
AreaMove() | Set the anchor point for a filled polygon. |
AreaDraw() | Add a new vertice to an area-fill polygon. |
AreaEnd() | Close and area-fill polygon, draw it and fill it. |
BNDRYOFF() | Turn off area-outline pen usage activated with SetOPen(). |
AreaCircle() | Draw a filled circle with a given radius and center point. |
AreaEllipse() | Draw a filled ellipse with the given radii and center point. |
Flood() | Flood fill a region starting at a given coordinate. |
RectFill() | Flood fill a rectangular area at a given location and size. |
Function | Description |
---|---|
BltClear() | Use the hardware blitter to clear a block of memory. |
SetRast() | Fill the RastPort.BitMap with a given color. |
ScrollRaster() | Move a portion of a RastPort.BitMap. |
BltPattern() | Draw a rectangular pattern of pixels into a RastPort.BitMap. The x-dimension of the rectangle must be word-aligned and word-sized. |
BltTemplate() | Draw a rectangular pattern of pixels into a RastPort.BitMap. The x-dimension of the rectangle can be arbitrarily bit-aligned and sized. |
BltBitMap() | Copy a rectangular area from one BitMap to a given coordinate in another BitMap. |
BltBitMapRastPort() | Copy a rectangular area from a BitMap to a given coordinate in a RastPort.BitMap. |
BltMaskBitMapRastPort() | Copy a rectangular area from a BitMap to a RastPort.BitMap through a mask bitplane. |
ClipBlit() | Copy a rectangular area from one RastPort to another with respect to their Layers. |
BitMapScale() | Scale a rectangular area within a BitMap to new dimensions. |
Function | Description |
---|---|
OwnBlitter() | Obtain exclusive access to the Amiga’s hardware blitter. |
DisownBlitter() | Relinquish exclusive access to the blitter. |
WaitBlit() | Suspend until the current blitter operation has completed. |
QBlit() | Place a bltnode-style asynchronous blitter request in the system queue. |
QBSBlit() | Place a bltnode-style asynchronous blitter request in the beam synchronized queue. |
CINIT() | Initialize the user Copper list buffer. |
CWAIT() | Instructs the Copper to wait for the video beam to reach a given position. |
CMOVE() | Instructs the Copper to place a value into a given hardware register. |
CBump() | Instructs the Copper to increment its Copper list pointer. |
CEND() | Terminate the user Copper list. |